[LeetCode] 2. Add Two Numbers 刷題筆記
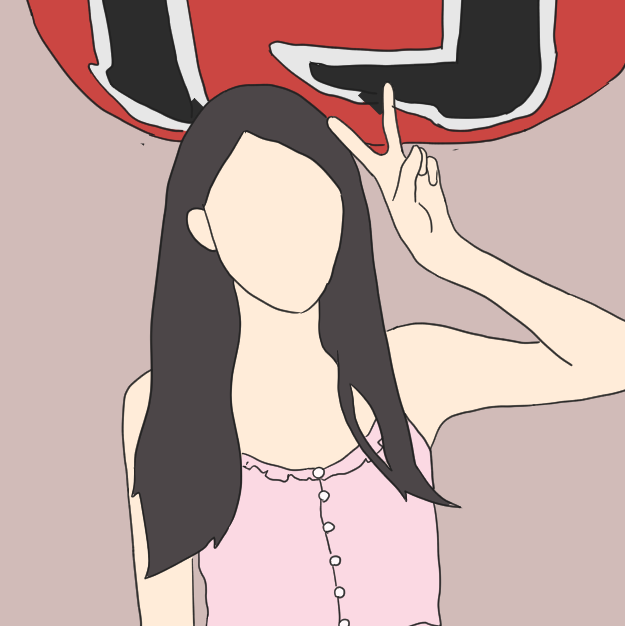
題目
LeetCode 題目連結:https://leetcode.com/problems/add-two-numbers/
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
輸入兩個不為空的 linked lists 代表兩個為非負數的整數,這些數字以反方向儲存,每一個 node 包含一個數字,將兩數相加,並回傳總和的 linked lists。
Example 1:
1 | Input: l1 = [2,4,3], l2 = [5,6,4] |
Example 2:
1 | Input: l1 = [0], l2 = [0] |
Example 3:
1 | Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9] |
解題過程
做法:
將l1
和l2
的.val
相加answers
裡,溢位的部分直接將answers.next
的值加1
,然後將l1
和l2
移到.next
,直到l1
和l2
皆等於null
。
1 | /** |
成績
Runtime: 128 ms (排名:89.43%)
Memory Usage: 43.8 MB (排名:85.30%)
心得
很久沒碰 linked lists 了,也沒用 javascript 寫過,一開始一直在想開頭會多一個 0,後來才想到直接回傳.next
就可以跳過開頭的 0 了。