[LeetCode] 7. Reverse Integer 刷題筆記
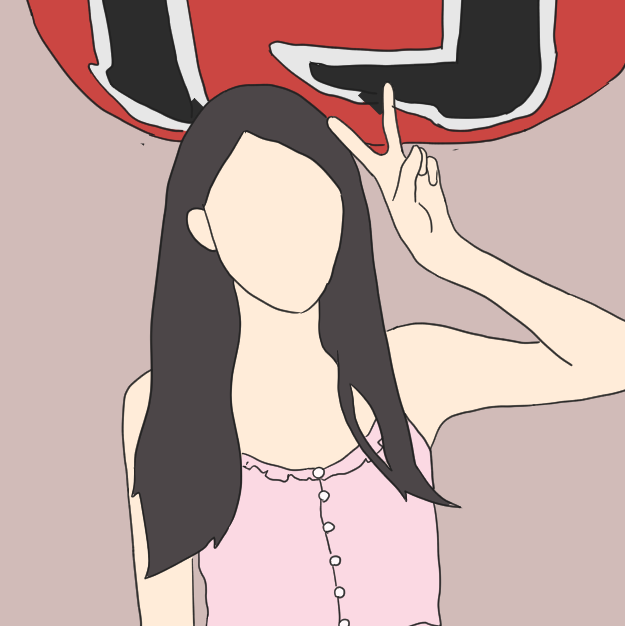
題目
Given a signed 32-bit integer x, return x with its digits reversed. If reversing x causes the value to go outside the signed 32-bit integer range [-231, 231 - 1], then return 0.
Assume the environment does not allow you to store 64-bit integers (signed or unsigned).
輸入一個 32-bit 的整數 x,將數字倒過來回傳。如果倒過來的數字超出[-2^31, 2^31 - 1]
範圍,則回傳 0。
Example 1:
1 | Input: x = 123 |
Example 2:
1 | Input: x = -123 |
Example 3:
1 | Input: x = 120 |
Example 4:
1 | Input: x = 0 |
解題過程
方法 1
基本上是把數字轉成字串,倒著
因為負號不需要倒轉,先取絕對值,將 x 轉成字串再返向存入一個新的字串,如果有負號最後把負號加回去。
1 | /** |
成績
Runtime: 100 ms (排名:58.87%)
Memory Usage: 40.5 MB (排名:35.38%)
方法 2
再思考怎麼改善的時候,突然想到或許不一定要用 string,直接用數字存也可以,於是就有了這個方法 2。
1 | /** |
成績
小小改善了一點
Runtime: 100 ms (排名:58.87%)
Memory Usage: 40.3 MB (排名:58.06%)